Bash + AWS CLI script to setup Terracotta AWS User + Read-only Policy
#!/bin/bash
# Define variables
USER_NAME="TerraformUser"
POLICY_NAME="TerraformReadOnlyAwsPolicy"
POLICY_DOCUMENT='{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": [
"aws-marketplace:Describe*",
"aws-marketplace:List*",
"aws-marketplace:Get*",
"cloudformation:Describe*",
"cloudformation:List*",
"cloudformation:Get*",
"cloudfront:Describe*",
"cloudfront:List*",
"cloudfront:Get*",
"cloudtrail:Describe*",
"cloudtrail:List*",
"cloudtrail:Get*",
"cloudwatch:Describe*",
"cloudwatch:List*",
"cloudwatch:Get*",
"dynamodb:Describe*",
"dynamodb:List*",
"dynamodb:Get*",
"ec2:Describe*",
"ec2:List*",
"ec2:Get*",
"iam:List*",
"iam:Get*",
"lambda:List*",
"lambda:Get*",
"rds:Describe*",
"rds:List*",
"rds:Get*",
"s3:List*",
"s3:Get*",
"s3:GetTags",
"s3:ListTags",
"s3:ListTagsForResource",
"sns:List*",
"sns:Get*",
"sqs:List*",
"sqs:Get*",
"sts:AssumeRole"
],
"Resource": "*"
}
]
}'
echo "Creating IAM user: $USER_NAME..."
aws iam create-user --user-name "$USER_NAME"
echo "Generating access keys for $USER_NAME..."
ACCESS_KEYS=$(aws iam create-access-key --user-name "$USER_NAME")
ACCESS_KEY_ID=$(echo "$ACCESS_KEYS" | jq -r '.AccessKey.AccessKeyId')
SECRET_ACCESS_KEY=$(echo "$ACCESS_KEYS" | jq -r '.AccessKey.SecretAccessKey')
echo "Creating IAM policy: $POLICY_NAME..."
POLICY_ARN=$(aws iam create-policy --policy-name "$POLICY_NAME" --policy-document "$POLICY_DOCUMENT" | jq -r '.Policy.Arn')
echo "Attaching policy to user..."
aws iam attach-user-policy --user-name "$USER_NAME" --policy-arn "$POLICY_ARN"
echo "IAM User and Policy setup completed."
echo "Access Credentials:"
echo "Access Key ID: $ACCESS_KEY_ID"
echo "Secret Access Key: $SECRET_ACCESS_KEY"
This script generates the user and policy necessary for Terracotta drift detection to work.
Pre-reqs
- must have
aws cli
installed locally and setup with credentials for the environment you want this in
touch <script>.sh
- Open your favorite text editor
- Copy/paste the code
chmod +X <script>.sh
- run script:
./<script>.sh
- Output example:
./terracottaCreateUserPolicy.sh
Creating IAM user: TerraformUserTest...
{
"User": {
"Path": "/",
"UserName": "TerraformUserTest",
"UserId": "AIDAXD6HNWBW5.....",
"Arn": "arn:aws:iam::<ORG>:user/TerraformUserTest",
"CreateDate": "2025-03-18T20:12:37+00:00"
}
}
Generating access keys for TerraformUserTest...
Creating IAM policy: TerraformReadOnlyAwsPolicyTest...
Attaching policy to user...
IAM User and Policy setup completed.
Access Credentials:
Access Key ID: AKIAXD6H.....
Secret Access Key: UrQOKKw3VPSUSRv.....
- Paste the access key and secret key into the UI and hit
Save Credentials
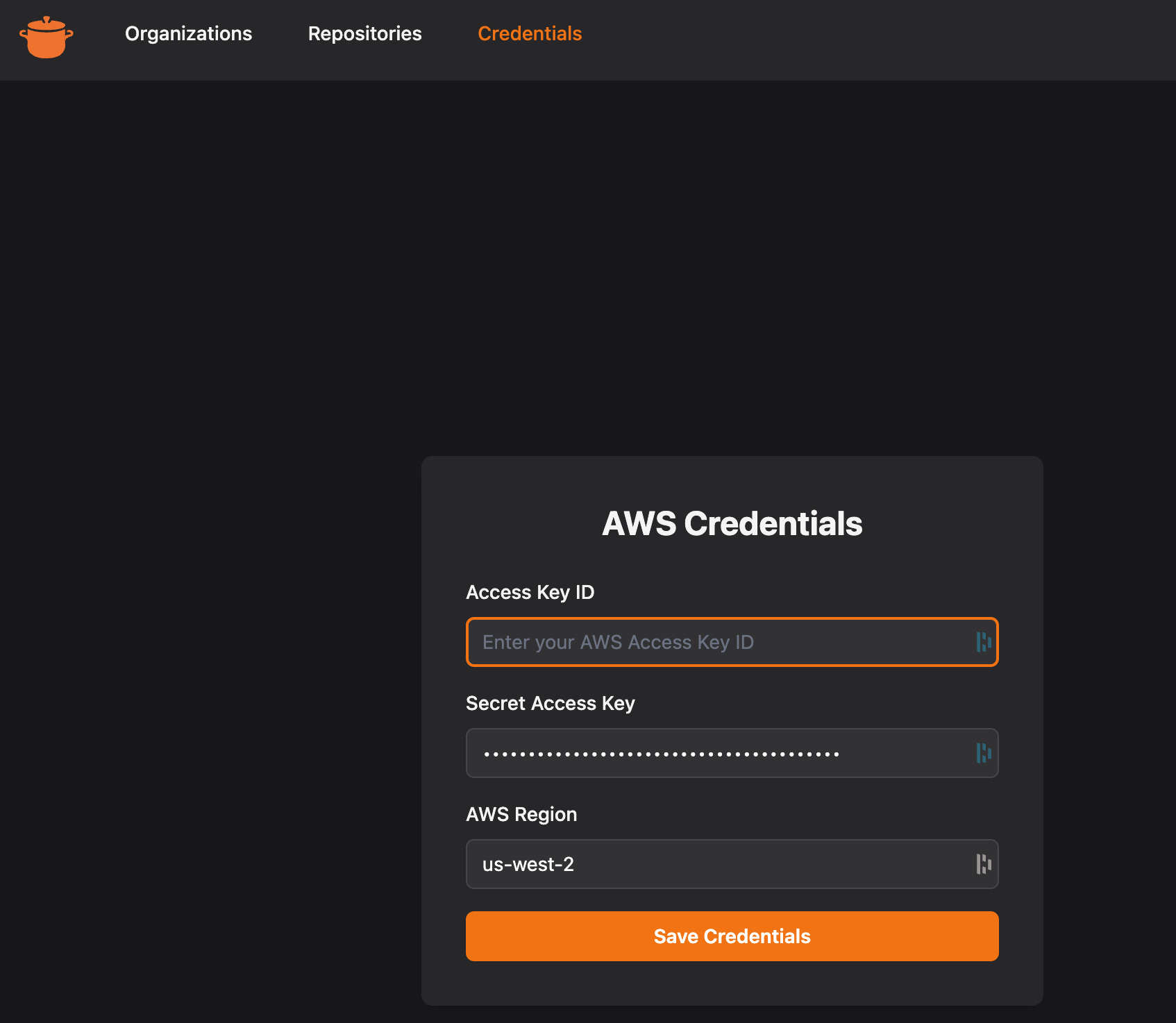
Updated 15 days ago